You Can Use The Printwriter Class To Open A File For Writing And Write Data To It.
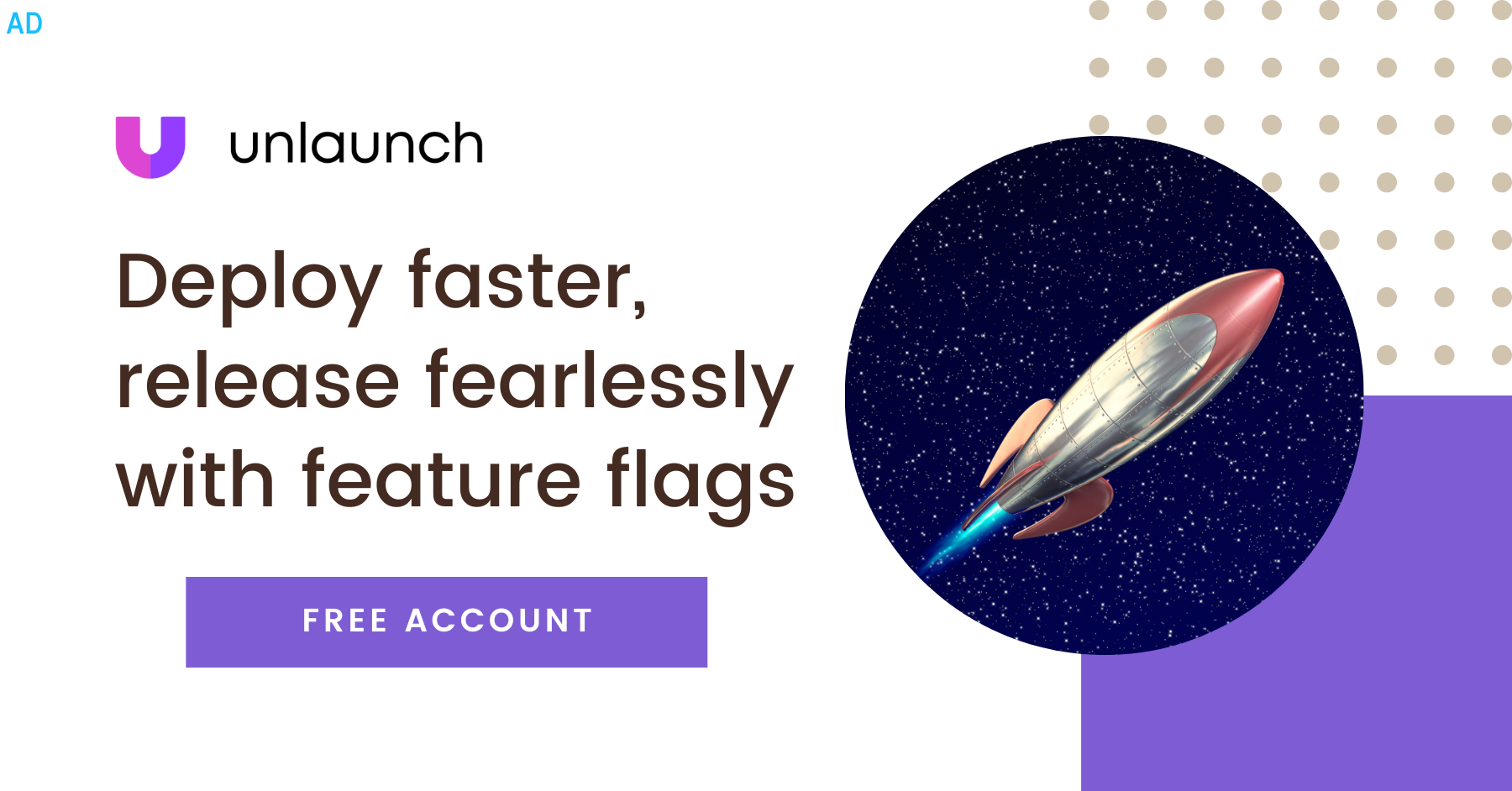
PrintWriter is a useful class in Java for writing formatted data to files or to text streams. By formatted data, I mean human readable value for data types like int
or boolean
as opposed to their byte representation (which is something you'd want if you are working with binary data such as images, jpegs, etc.) In this tutorial, we'll look at examples and learn how to use PrintWriter
to write data to files. Let's get started.
PrintWriter
a subclass of java.io.Writer, which is an abstract class for writing to character streams. PrintStream
can be used to write formatted data to any character stream. It's most commonly used for writing data to human readable text files or reports.
In our first example, we'll use PrintWriter
to create a new file by passing in the filename to its constructor as a string. If the file doesn't exists, it will be created. If it already exists, it will be overwritten and all its contents will be lost. Once we have opened the file, we'll use PrintWriter.print(...)
, and PrintWriter.println(...)
methods to write different data types: int
, String
and boolean
to the file.
package com.codeahoy.ex ; import java.io.FileNotFoundException ; import java.io.PrintWriter ; public class Main { public static void main ( String [] args ) { try { PrintWriter printWriter = new PrintWriter ( "out_codeahoy.txt" ); printWriter . print ( 123 ); printWriter . print ( " This is a normal string " ); printWriter . print ( false ); printWriter . close (); } catch ( FileNotFoundException fnf ) { fnf . printStackTrace (); } } }
After running this program, a new file called out_codeahoy.txt
is created in the project directory. If you open it, it should contain the following:
123 This is a normal string false
The next example shows how to use PrinterWriter
to write to another Writer
subclass: java.io.FileWriter. Let's see how it works.
package com.codeahoy.ex ; import java.io.FileNotFoundException ; import java.io.FileWriter ; import java.io.IOException ; import java.io.PrintWriter ; public class Main { public static void main ( String [] args ) { try { FileWriter fileWriter = new FileWriter ( "out_codeahoy2.txt" ); PrintWriter printWriter = new PrintWriter ( fileWriter ); printWriter . print ( 12345 ); printWriter . print ( " This is a string " ); printWriter . print ( true ); printWriter . close (); } catch ( IOException ioe ) { ioe . printStackTrace (); } } }
After we run the program above, it will create a new file out_codeahoy2.txt
with the following contents:
12345 This is a string true
Before I end this post, here are all the constructors for the PrintWriter
class. You can see that it supports a variety of different types as its arguments.
- PrintWriter(File file): Creates a new PrintWriter, without automatic line flushing, with the specified file.
- PrintWriter(File file, String csn): Creates a new PrintWriter, without automatic line flushing, with the specified file and charset.
- PrintWriter(OutputStream out): Creates a new PrintWriter, without automatic line flushing, from an existing OutputStream.
- PrintWriter(OutputStream out, boolean autoFlush): Creates a new PrintWriter from an existing OutputStream.
- PrintWriter(Writer out): Creates a new PrintWriter, without automatic line flushing.
- PrintWriter(Writer out, boolean autoFlush): Creates a new PrintWriter.
- PrintWriter(String fileName): Creates a new PrintWriter, without automatic line flushing, with the specified file name.
- PrintWriter(String fileName, String csn): Creates a new PrintWriter, without automatic line flushing, with the specified file name and charset.
That's all. Hope you enjoyed.
If you like this post, please share using the buttons above. It will help CodeAhoy grow and add new content. Thank you!
You Can Use The Printwriter Class To Open A File For Writing And Write Data To It.
Source: https://codeahoy.com/java/PrintWriter-JI_10/
Posted by: hansenmirere.blogspot.com
0 Response to "You Can Use The Printwriter Class To Open A File For Writing And Write Data To It."
Post a Comment